In this tutorial, we will learn how to use Flake8 to ensure Python code quality and Black to enforce consistent style. These two tools complement each other perfectly—Flake8 helps you identify common mistakes and ensures your code follows good practices, while Black automatically formats your code for consistency and readability.
Purpose
Integrating Flake8 and Black in Python projects ensures code quality and consistent style. Flake8 checks for errors and enforces best practices, while Black formats code automatically, keeping it clean and readable.
Scope
This applies to any Python project that needs automatic code checks, error detection, and style consistency. It helps developers better code, avoid style debates, and integrate quality checks into the development workflow.
Why Use Flake8 and Black?
- Flake8 checks your code for issues like syntax errors, unused variables, and PEP 8 violations. It ensures your code follows best practices.
- Black formats your code in a consistent style. It saves you time by automatically adjusting line breaks, indentation, and other formatting rules, ensuring that the style remains consistent across your project.
Step-by-Step Guide
Step 1: Install Flake8 and Black
First, let’s install both Flake8 and Black using pip
:
pip install flake8 black
Step 2: Run Flake8 to Check Code Quality
Once Flake8 is installed, you can run it to check your Python code for potential issues.
To check a single Python file:
flake8 your_script.py
To check an entire directory:
flake8 /path/to/your/project
Flake8 will scan your code for:
- PEP 8 violations (e.g., incorrect indentation, line lengths)
- Syntax errors (e.g., missing colons, undefined variables)
- Unused imports or variables
Sample flake8 generated output with issues found along with line numbers, making it easy to locate and fix them:
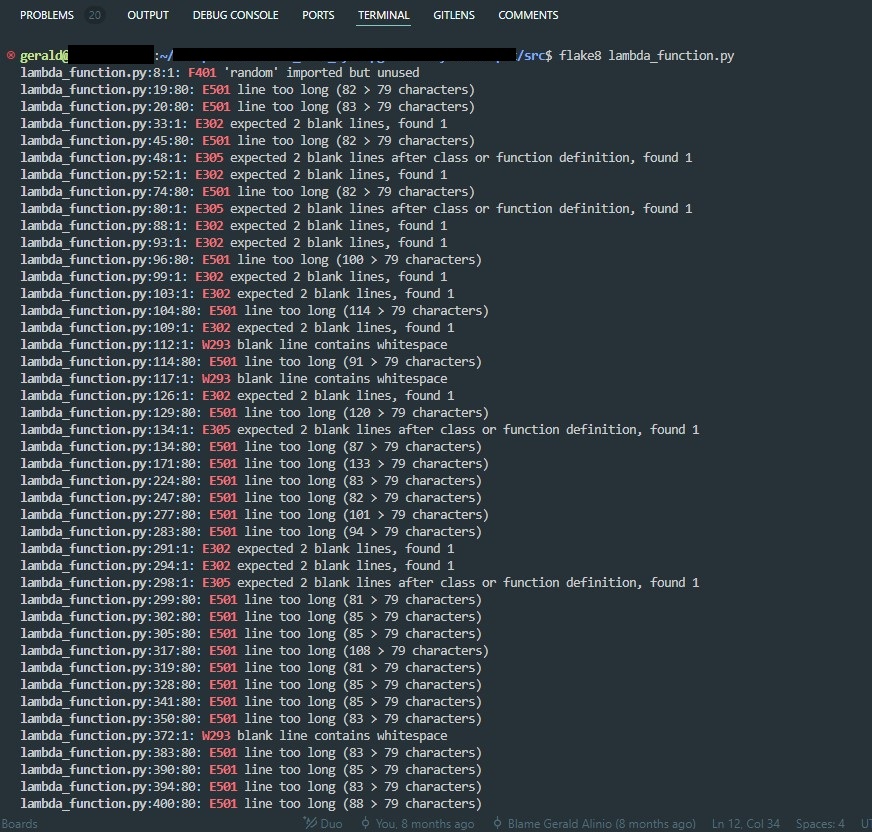
Step 3: Configure Flake8 (Optional)
You can customize Flake8 to match your project’s style guide by creating a .flake8
configuration file or adding rules in setup.cfg
. Here’s an example .flake8
file:
[flake8] max-line-length = 88 ignore = E203, W503
max-line-length = 88
tells Flake8 to allow lines up to 88 characters, which is the default for Black.ignore = E203, W503
disables some rules that conflict with Black‘s formatting.
Step 4: Run Black to Format Your Code
After ensuring your code has no major issues with Flake8, use Black to format your code for consistent style.
To format a single Python file:
black your_script.py
To format an entire directory:
black /path/to/your/project
Black will automatically reformat your code according to PEP 8 standards, but with its own rules (like using 88-character lines instead of 79).
Below sample output generated by Black after fixing unformatted line code issues and more:
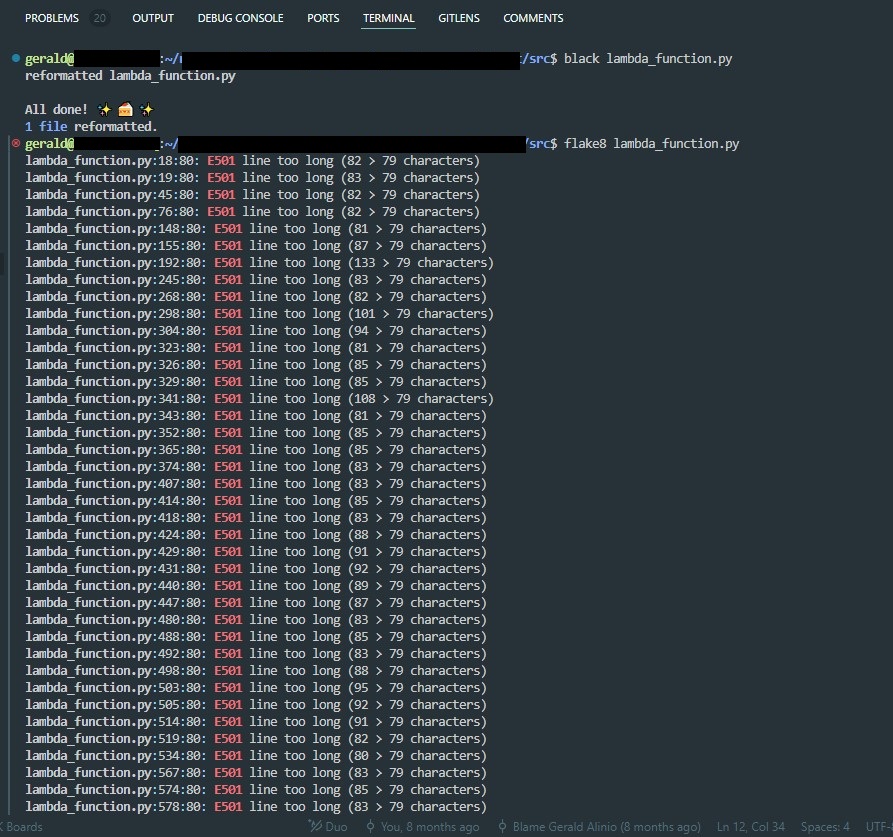
Step 5: Check Formatting without Making Changes
If you want to check if your code is formatted according to Black, without actually modifying the files, use:
black --check your_script.py
If the file isn’t formatted properly, Black will return a non-zero exit code.
Sample output when Black fails to format the file due to a syntax issue:
error: cannot format lambda_function.py: Cannot parse: 1304:13: )test Oh no! 💥 💔 💥 1 file would fail to reformat.
So you need to investigate this issue first using Flake8 before performing Black command.
Step 6: Automate with Pre-Commit Hooks (Optional)
You can set up Flake8 and Black to run automatically before committing your code to version control (like Git). This helps ensure your code is always clean and consistent before merging.
First, install pre-commit
:
pip install pre-commit
Next, create a .pre-commit-config.yaml
file in your project directory:
repos: - repo: https://github.com/PyCQA/flake8 rev: 7.1.1 hooks: - id: flake8 - repo: https://github.com/psf/black rev: 24.10.0 hooks: - id: black
Install the pre-commit hook:
pre-commit install
Now, Flake8 and Black will automatically run every time you try to commit code, ensuring that your code meets both quality and style standards.
Example command to run it manually for a specific file:
pre-commit run --files test.py
You can disable this tool manually by renaming the pre-commit
hook file in the .git/hooks
directory. For example, you can rename the file to pre-commit.disabled
:
mv .git/hooks/pre-commit .git/hooks/pre-commit.disabled
This will effectively disable the hook. To re-enable it, simply rename it back to pre-commit
.
For more details on how to install pre-commit tools, also check out their official documentation or explore available configurations and hooks that can improve your development workflow.
Step 7: Combine Flake8 and Black for Maximum Efficiency
When using both Flake8 and Black together, follow these steps:
- Run Flake8 first to catch any quality issues in your code, such as unused imports, logical errors, or syntax problems:
flake8 your_script.py
- Run Black next to automatically format your code, making sure it’s clean and follows consistent style rules:
black your_script.py
By running these tools in this order, you can ensure that your code is both high-quality and consistently styled.
Summary of Key Commands
- Install Flake8 and Black:
pip install flake8 black
- Check code quality with Flake8:
flake8 your_script.py
- Automatically format code with Black:
black your_script.py
- Check formatting without changing code:
black --check your_script.py
- Set up pre-commit hooks:
- Add the configuration to
.pre-commit-config.yaml
and runpre-commit install
- Add the configuration to
- Run all hooks for specific file:
pre-commit run --files test.py
Conclusion
By using Flake8 for code quality checks and Black for automatic formatting, you can ensure that your Python code is clean, consistent, and easy to maintain. These tools save time, reduce errors, and remove debates over code style, letting you focus on writing functional code.